CIS 371 |
Simple Web Server |
Winter 2023 |
https://classroom.github.com/a/Xj0tUN7v
This video demonstrates how to use GitHub Classroom with this assignment.
Objective
Learn the inner workings of a basic web server to better understand what underlies web platforms. In particular, students will observe the details of- interpreting an incoming request,
- identifying and finding the requested resource,
- preparing the proper response (which may be an error message), and
- delivering the content (which could be either text or binary data).
Details
Your web server must:
- Listen on port 8534 by default. (You can make this configurable, if you like; but, 8534 needs to be the default.)
- Correctly serve text, html, pdf, and images (gif, png, and jpeg). You may support additional types if you like.
- You response headers must include
Content-Type
andContent-Length
.- You may assume the content type from the file's extension.
- You may hard-code the file extension to mime-type mapping.
- If there is no file extension, or if the file extension not recognized, use "
text/plain
"
- Return a 404 error when appropriate. (The response should also include a brief HTML message describing the problem.)
- Return a 400 error message when appropriate. (The response should also include a brief HTML message describing the problem.)
- If the requested document is a directory:
- Return
index.html
, if present. - Otherwise, return a simple directory listing (with links). Important: Your link to
subdirectories must end with '
/
', otherwise, the web browser won't properly reset the base directory. (In other words, yourhref
needsImages/
not justImages
.)
- Return
Challenges
- Make your server multi-threaded so that it can serve multiple requests at once.
- Implement an HTTPS server. Warning: I hear that getting the certificates set up correctly can be challenging. I've never done this; so, if you run into trouble, I'll be of limited help.
Rules
- You may work on this assignment in teams of at most two.
- You may use any language you like. Realize, however, that I will be of limited help if you choose something
other than Java. (I know C, C++, and Ruby well; but, I don't know much about the particular networking
libraries.
I do not know any
.net
languages. I do not know Windows well.) - You may use standard socket and I/O libraries. You may not use any libraries that implement significant
web server functionality (e.g.,
com.sun.net.httpserver
). - Cite any sources you consult. Avoid articles that provide complete (or nearly complete) solutions.
- Every file should begin with a comment that includes the names of all team members.
- Follow good design practices. In particular, break your code into methods to avoid code duplication and to keep methods from getting too long.
Submission and Grading
This project will be partially auto-tested using GitHub Actions. Begin by using following this link to create a repository for this project. Once you have created your repository for this assignment, clone the repo to your local machine. Your repository will contain the following files:
MyStaticWebServer.java
- This file contains some code to help you get started (including most of the code from the sample server we
discussed in class). You don't have to use this file, or any of the code in it. But, if you choose a
different file, you will have to adjust the
Makefile
accordingly so that GitHub can build and run your server. (I'm happy to help you do this.) Makefile
- GitHub will use this file to build, run, and test your server. If you put all your code into
MyStaticWebServer.java
, you won't need to modify this makefile. However, if you have a different design in mind, go ahead. I'm happy to help you adjust the makefile as necessary. .github
- Configuration files for GitHub Actions. Don't mess with files here. (Attempting to modify the testing process to either (1) create a "false pass", or (2) examine the test cases is a serious violation of the CIS Academic Honesty Policy.)
studentData
- This directory contains files you can use to test your server. To launch your server with this directory as
the root,
cd
into this directory- Run java -cp .. MyStaticWebServer
testPlan.txt
- Complete this document to specify how you will test your server.
Steps for completion and submission
- Begin by writing your test cases. Look through the requirements above, and write a short paragraph describing
how you will verify that your server meets each requirement. (
testPlan.txt
includes a couple samples.) - Write your code. Make sure your name appears in all files.
- When your code passes all of your tests, push to the
master
branch. - Go to the
Actions
tab on the GitHub page for this project and launch the tests. - If your code fails my tests:
- Figure out what is missing from your test plan and add it.
- Fix your code
- Resubmit.
- When your code passes the GitHub tests, schedule a demo with me.
Hints and Reminders
- The URL for a directory should end with a '
/
', otherwise the relative links to the files won't work correctly. (See details above.) - Remember to call
close
on your socket at the end of every call. Forgetting to close a socket may cause the next request to hang. - You must have a written test plan (described above).
- This project will not be graded until it passes all of my tests. Your grade is based primarily on when you get all the automated tests passing. (Code design and testing will also factor into your grade.)
- There are two stages to passing the tests.
- First, your code must pass the automated tests in GitHub
- Then, you must demonstrate your server to me and demonstrate that it can properly handle requests from a standard web browser
- Every file should include all team member names.
Updated Monday, 20 February 2023, 6:05 PM
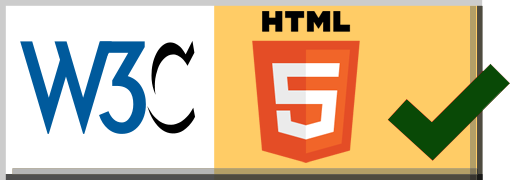